Understanding Bubble Sort and Insertion Sort Algorithms in C: Explained with Code
Bubble Sort Algorithm
Bubble Sort is the simplest sorting algorithm that works by repeatedly swapping the adjacent elements if they are in the wrong order. This algorithm is not suitable for large data sets as its average and worst-case time complexity is quite high.
Explanation:
In Bubble Sort algorithm,
- Traverse from left and compare adjacent elements and the higher one is placed at the right side.
- In this way, the largest element is moved to the rightmost end at first.
- This process is then continued to find the second largest and place it and so on until the data is sorted.
How does Bubble Sort Work?
Let us understand the working of bubble sort with the help of the following illustration:
Input:
arr[] = {6, 3, 0, 5}
First Pass:
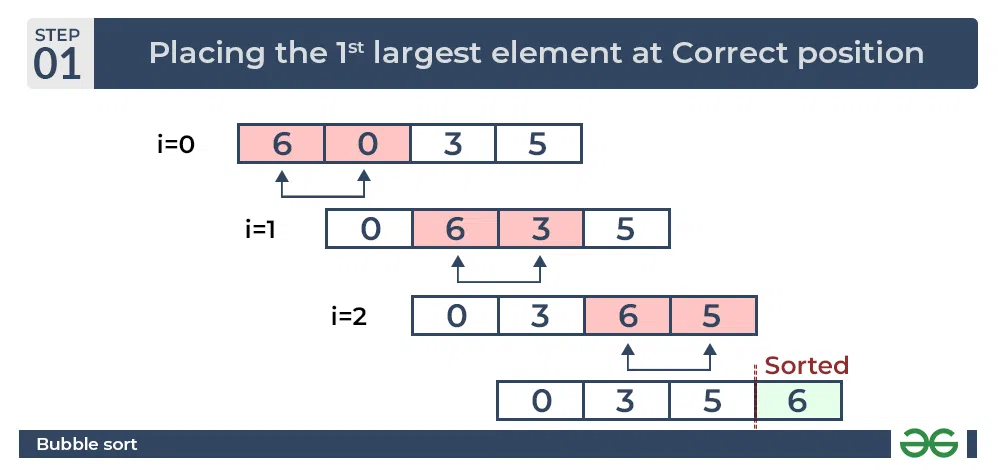
Second Pass:
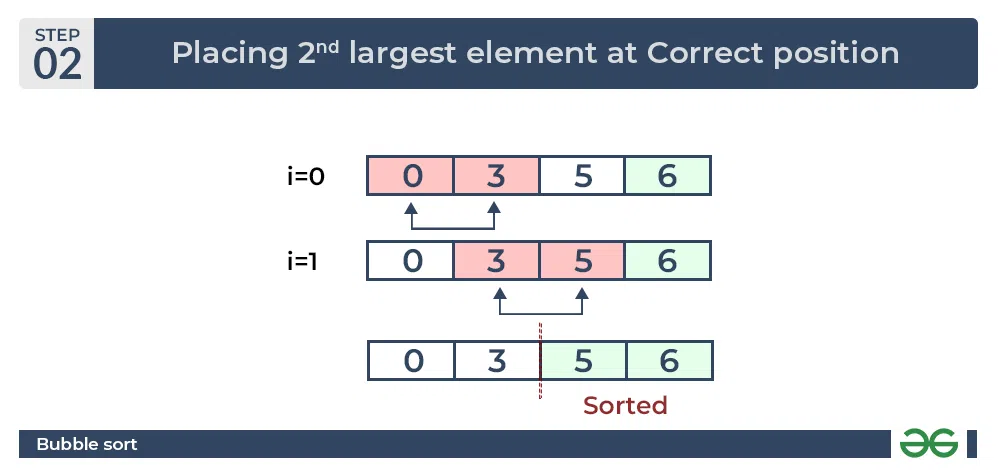
Third Pass:
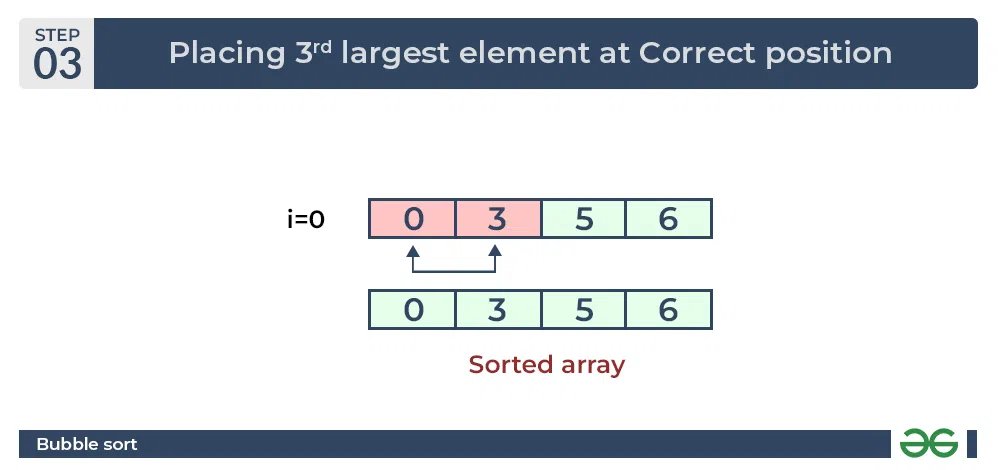
Total no. of passes:
n-1
Total no. of comparisons:
n*(n-1)/2
Implementation of Bubble Sort
Let's break down the provided C code:
printarray Function:
This function prints the elements of an array.
void printarray(int* A, int n){
for(int i = 0; i < n; i++){
printf("%d ", A[i]); // Print each element of the array
}
printf("\n"); // Move to the next line after printing all elements
}
bubblesort Function:
This function implements the Bubble Sort algorithm to sort the array.
void bubblesort(int* A, int n){
int temp; // Temporary variable for swapping elements
// Outer loop for the number of passes required to sort the array
for(int i = 0; i < n - 1; i++){
// Inner loop for the number of comparisons per pass
for(int j = 0; j < n - 1 - i; j++){
// Compare adjacent elements and swap if necessary
if(A[j] > A[j + 1]){
temp = A[j]; // Store the value of A[j] in temp
A[j] = A[j + 1]; // Assign the value of A[j+1] to A[j]
A[j + 1] = temp; // Assign the value of temp to A[j+1]
}
}
}
}
Main Function:
This is the main function where we declare an array, sort it using Bubble Sort, and print the sorted array.
int main(){
int A[] = {12, 54, 65, 7, 23, 9}; // Declare and initialize an integer array
int n = 6; // Number of elements in the array
// Print the array before sorting
printf("The array before sorting: ");
printarray(A, n);
// Sort the array using the bubble sort algorithm
bubblesort(A, n);
// Print the array after sorting
printf("The array after sorting: ");
printarray(A, n);
return 0; // Return 0 to indicate successful execution
}
Conclusion:
In this article, we learned how to implement the Bubble Sort algorithm in C to sort an array of integers. Understanding sorting algorithms is essential for any programmer, as sorting is a common task in many applications.